Super keyword
The super keyword is used in a derived class to refer to the members of the parent class.
- When class members are overridden in the derived class, It is necessary to use the super keyword to access members of a parent class
- With super keyword, it is allowed to access the class members of parent class which is only one level up
If the method of the parent class is overridden in the child class, then using the ‘super’ keyword parent class method can be accessed from the child class.
Super keyword example
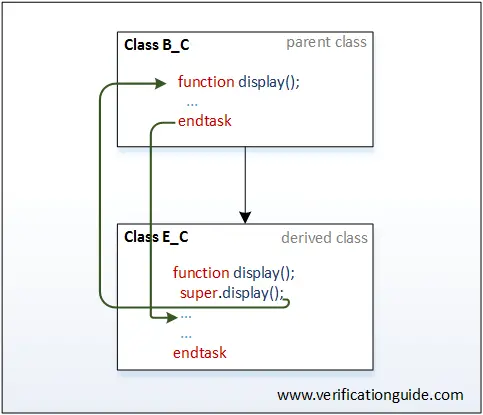
In below example,
The parent class has the method ‘display’.
Implementing the method display in the child class will override the parent class method.
By calling super.display() from child class, the display method of the parent class can be accessed.
class parent_class; bit [31:0] addr; function display(); $display("Addr = %0d",addr); endfunction endclass class child_class extends parent_class; bit [31:0] data; function display(); super.display(); $display("Data = %0d",data); endfunction endclass module inheritence; initial begin child_class c=new(); c.addr = 10; c.data = 20; c.display(); end endmodule
Simulator Output
Addr = 10 Data = 20
The below example is by removing the super.display() from the display method of the child class.
class parent_class; bit [31:0] addr; function display(); $display("Addr = %0d",addr); endfunction endclass class child_class extends parent_class; bit [31:0] data; function display(); $display("Data = %0d",data); endfunction endclass module inheritence; initial begin child_class c=new(); c.addr = 10; c.data = 20; c.display(); end endmodule
Simulator Output
Data = 20