SystemC supports all C++ data types; in addition SystemC provides additional data types for describing hardware.Data types are explained in two parts,
- C++ data types
- SystemC data types
C++ Data Types
Table of Contents
- Fundamental datatypes
- Additional datatypes
Fundamental Data Types:
Data Types
|
Keyword
|
Description
|
Character types
|
char
|
character with width of 8bits
|
wchar_t
|
Represents the largest character set
|
|
Integer types
|
int
|
integer with width of 32 bits
|
signed int
|
signed integer with width of 32 bits, values can be
+ve/-ve/’0’ |
|
unsigned int
|
unsigned integer with width of 32 bits, values must be
+ve/’0’ |
|
short
|
Short integer with width of 16 bits
|
|
signed short
|
signed short integer with width of 16 bits, values can
be +ve/-ve/’0’ |
|
unsigned short
|
Unsigned Short integer with width of 16 bits, values
must be +ve/’0’ |
|
long
|
Long integer with width of 32 bits
|
|
signed long
|
Signed long integer with width of 32 bits, values can
be +ve/-ve/’0’ |
|
unsigned long
|
Unsigned long integer with width of 32 bits, values
must be +ve/’0’ |
|
Floating-point types
|
float
|
Floating point number with width of 32bits.
|
double
|
Double precision floating point number with width of
64bits. |
|
long double
|
Long precision floating point number with width of
64bits. |
|
Boolean type
|
bool
|
Boolean type with size of 8bits,it can take values
‘0’/’1’ |
Void type
|
void
|
With no value
|
Declaration:
Example-1: Initialization and Declaration
#include "systemc.h" int sc_main (int argc, char* argv[]) { //variable declaration int a; float b; float c; //initialization a = 10; b = 20.5; //addtion c = a + b; cout <<"Value of a = "<< c <<endl; // Terminate simulation return 0; }
Simulator Output:
Example-2: Declaration and Initialization
#include "systemc.h" int sc_main (int argc, char* argv[]) { //variable declaration and initialization //variables can be initialized in three different ways as shown below int a=10; int b(1.3); float c{2.8}; cout <<"Value of a = "<< a <<endl; cout <<"Value of b = "<< b <<endl; cout <<"Value of c = "<< c <<endl; // Terminate simulation return 0; }
Simulator Output:
Value of b = 1
Value of c = 2.8
Execute the above code on
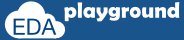
Example-3: Initializing char
#include "systemc.h" int sc_main (int argc, char* argv[]) { //variable declaration and initialization char a[] = "Hello world"; char b[5]; //initialization b[0] = 'H'; b[1] = 'e'; b[2] = 'l'; b[3] = 'l'; b[4] = 'o'; //below initializations types are not allowed //b = "Hello"; //b = {'H','e','l','l','o'}; cout <<"Value of a = "<< a <<endl; cout <<"Value of b = "<< b <<endl; // Terminate simulation return 0; }
Simulator Output:
Additional Data Types:
- String Data Types
- Enumerated Data Types
- Typedef Data Types
String Types:
As Fundamental data types, we cant use the string data type directly. To use string data type need to import the string header standard library.( #include <string>)
Example-4: enum example
#include "systemc.h" #include <string> using namespace std; int sc_main (int argc, char* argv[]) { //string declaration and initialization string mystring = "Hello world"; string mystring_1; string mystring_2; string mystring_3; //initialization, can be initialized in three ways. mystring_1 = "1. I am String in C++/SystemC"; mystring_2 = ("2. I am String in C++/SystemC"); mystring_3 = {"3. I am String in C++/SystemC"}; cout <<"mystring = "<< mystring <<endl; cout <<"mystring_1 = "<< mystring_1 <<endl; cout <<"mystring_2 = "<< mystring_2 <<endl; cout <<"mystring_3 = "<< mystring_3 <<endl; // Terminate simulation return 0; }
Simulator Output:
An enumerated type defines a set of named values. The simplest enumerated type declaration contains a list of constant names and one or more variables.enum type_name {value1,value2,…,valuen} object_names;
This creates the type type_name, which can take any of value1, value2, … ,valuen as value. Variables of this type can directly be instantiated as object_names.
In the following example, colors is defined to be variable of the Colors type that includes the members red, green, blue, yellow, white, black.
enum Colors { red, green, blue, yellow, white, black } Color_t;
Color_t can be used directly used as variable, but Colors is used as type to declare the variables.
Example-5: Enum Example
#include "systemc.h" int sc_main (int argc, char* argv[]) { //declaration enum Colors { red, green, blue, yellow, white, black } Colors_t; Colors my_color; Colors_t = white; my_color = blue; cout <<"Colors_t = "<< Colors_t <<endl; cout <<"mystring = "<< my_color <<endl; // Terminate simulation return 0; }
Simulator Output:
The actual values are default to integers starting at 0 and then increase. in the above example by default variable will get default value of 0,1,2,3,4,5 respectively from red.
The values can be set for the names and also values can be set for some of the names and not set for other names. A name without a value is automatically assigned an increment of the value of the previous name.
In the following example value is set for green = 2, yellow = 8.
enum Colors { red, green=2, blue, yellow=8, white, black };
Example-6: Enum Example
#include "systemc.h" int sc_main (int argc, char* argv[]) { //declaration enum Colors { red, green=2, blue, yellow=8, white, black }; Colors my_color; my_color = blue; cout <<"my_color value is = "<< my_color <<endl; my_color = black; cout <<"my_color value is = "<< my_color <<endl; // Terminate simulation return 0; }
Simulator Output:
Constant Expressions:
Constant values can be named by using const keyword.
Example-1: Constant
#include "systemc.h" const int seconds_per_minute = 60; int sc_main (int argc, char* argv[]) { cout <<"1 minute = "<< seconds_per_minute << " seconds"<<endl; cout <<"8 minute = "<< 8*seconds_per_minute << " seconds"<<endl; // Terminate simulation return 0; }
Simulator Output:
1 minute = 60 seconds
8 minute = 480 seconds
Execute the above code on
❮ Previous Next ❯