SystemVerilog Inheritance
Table of Contents
SystemVerilog Inheritance. Inheritance is an OOP concept that allows the user to create classes that are built upon existing classes. The new class will be with new properties and methods along with having access to all the properties and methods of the original class. Inheritance is about inheriting base class members to the extended class.
- New classes can be created based on existing classes, this is referred to as class inheritance
- A derived class by default inherits the properties and methods of its parent class
- An inherited class is called a subclass of its parent class
- A derived class may add new properties and methods, or modify the inherited properties and methods
- Inheritance allows re-usability. i.e. derived class by default includes the properties and methods, which is ready to use
- If the class is derived from a derived class, then it is referred to as Multilevel inheritance
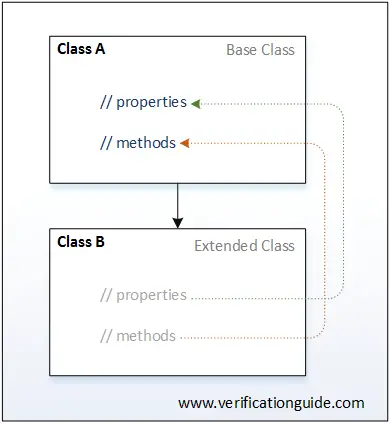
Inheritance Terminology
Parent Class
- It’s an existing class;
- The class whose features are inherited
- The parent class is also known as a base class, superclass
Child Class
- It’s an extended class;
- The class that inherits the other class is known as subclass
- The child class is also known as an extended class, derived class, subclass
Inheritance Example
Parent class properties are accessed using child class handle, i.e child class will have (inherit) parent class properties and methods.
In the below example,
parent_class is base class and child_class is written by extending the parent_class.
so child_class is derived from a base class, and it inherits the properties of the parent class.
Though the addr is not declared in child_class, it is accessible. because it is inherited from the parent class.
class parent_class; bit [31:0] addr; endclass class child_class extends parent_class; bit [31:0] data; endclass module inheritence; initial begin child_class c = new(); c.addr = 10; c.data = 20; $display("Value of addr = %0d data = %0d",c.addr,c.data); end endmodule
Simulator Output
Value of addr = 10 data = 20