do while loop
Table of Contents
A do while loop is a control flow statement that allows code to be executed repeatedly based on a given condition.
do while loop syntax
do begin // statement -1 ... // statement -n end while(condition);
In do-while,
- the condition will be checked after the execution of statements inside the loop
- the condition can be any expression.
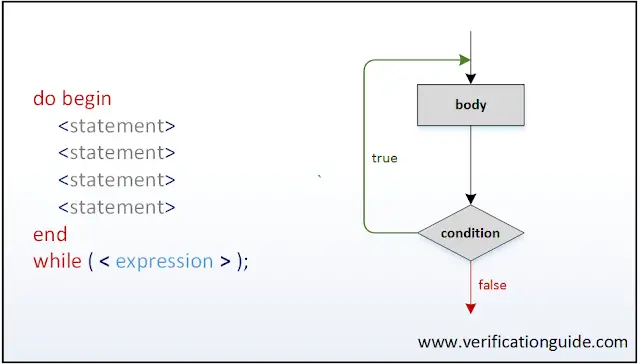
do-while is similar to while loop but in case of while loop execution of statements happens only if the condition is true. In a do while, statements inside the loop will be executed at least once even if the condition is not satisfied.
do while loop example
module do_while; int a; initial begin $display("-----------------------------------------------------------------"); do begin $display("\tValue of a=%0d",a); a++; end while(a<5); $display("-----------------------------------------------------------------"); end endmodule
Simulator output:
----------------------------------------------------------------- Value of a=0 Value of a=1 Value of a=2 Value of a=3 Value of a=4 -----------------------------------------------------------------
do while loop example 2
module do_while; int a; initial begin $display("-----------------------------------------------------------------"); do begin $display("\tValue of a=%0d",a); a++; end while(a>5); $display("-----------------------------------------------------------------"); end endmodule
Simulator Output
----------------------------------------------------------------- Value of a=0 -----------------------------------------------------------------
while loop SystemVerilog
A while loop is a control flow statement that allows code to be executed repeatedly based on a given condition.
while loop syntax
while(condition) begin // statement -1 ... // statement -n end
In a while,
- Execution of statements within the loop happens only if the condition is true
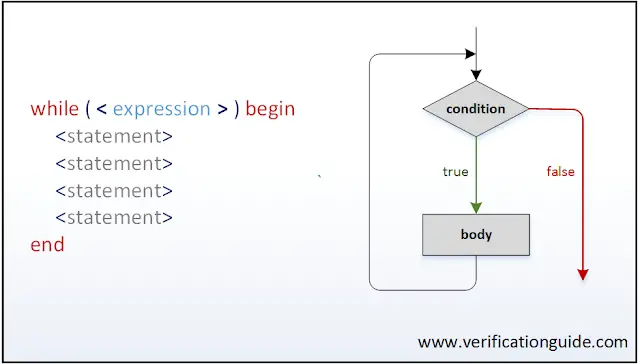
while loop example
module while_loop; int a; initial begin $display("-----------------------------------------------------------------"); while(a<5) begin $display("\tValue of a=%0d",a); a++; end $display("-----------------------------------------------------------------"); end endmodule
Simulator Output
----------------------------------------------------------------- Value of a=0 Value of a=1 Value of a=2 Value of a=3 Value of a=4 -----------------------------------------------------------------
while loop example 2
module while_loop; int a; initial begin $display("-----------------------------------------------------------------"); while(a>5) begin $display("\tValue of a=%0d",a); a++; end $display("-----------------------------------------------------------------"); end endmodule
Simulator Output
----------------------------------------------------------------- -----------------------------------------------------------------