Nonblocking TLM port and Imp Port
Table of Contents
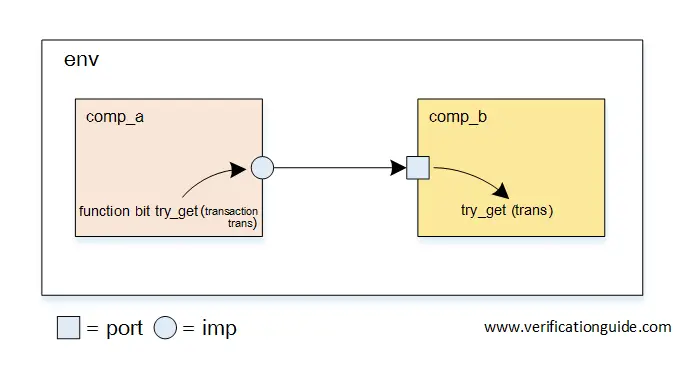
This example shows how to declare, create and connect the TLM non-blocking get ports, implementing and using the TLM non-blocking get methods. In this example blocking ports of the previous example ( TLM Get ports and imp_ports ) are replaced with the non-blocking get ports. It is preferred to refer to TLM Get ports and imp_ports example before going through this example.
TLM TesetBench Components are,
———————————————————-
Name Type
———————————————————-
uvm_test_top basic_test
env environment
comp_a component_a
trans_out uvm_nonblocking_get_imp
comp_b component_b
trans_in uvm_nonblocking_get_port
———————————————————-
Declare and Create TLM Get Port in comp_b
class component_b extends uvm_component; transaction trans; //Step-1. Declaring the get port uvm_nonblocking_get_port# ( transaction ) trans_in; `uvm_component_utils( component_b ) //--------------------------------------- // Constructor //--------------------------------------- function new ( string name , uvm_component parent ); super . new ( name , parent ); trans_in = new ( "trans_in" , this ); //Step-2. Creating the port endfunction : new //--------------------------------------- // run_phase //--------------------------------------- virtual task run_phase ( uvm_phase phase ); phase.raise_objection ( this ); `uvm_info(get_type_name(),$sformatf( " Requesting transaction." ) , UVM_LOW ) `uvm_info(get_type_name(),$sformatf( " Before calling port get method" ) , UVM_LOW ) //Step-3. Calling try_get to receive the transaction if ( trans_in.try_get ( trans ) ) begin //{ `uvm_info(get_type_name(),$sformatf( " recived transaction from get method" ) , UVM_LOW ) `uvm_info(get_type_name(),$sformatf( " Printing trans, \n %s" , trans.sprint ( ) ) , UVM_LOW ) end //} else `uvm_info(get_type_name(),$sformatf( " Not recived transaction from get method" ) , UVM_LOW ) `uvm_info(get_type_name(),$sformatf( " After calling port get method" ) , UVM_LOW ) phase.drop_objection ( this ); endtask : run_phase endclass : component_b |
try_get() method is used to receive the transaction form comp_b. try_get() methods returns ‘1’ on successful receive of transaction, otherwise ‘0’.
Declare and Create TLM Imp_port in comp_a
class component_a extends uvm_component; uvm_nonblocking_get_imp# ( transaction , component_a ) trans_out; //Step-1: Declare get Imp_port `uvm_component_utils( component_a ) //--------------------------------------- // Constructor //--------------------------------------- function new ( string name , uvm_component parent ); super . new ( name , parent ); trans_out = new ( "trans_out" , this ); //Step-2. Create the port endfunction : new //--------------------------------------- // Imp port try_get method //--------------------------------------- //Step-3. try_get method to send trans to comp_b virtual function bit try_get ( output transaction trans ); `uvm_info(get_type_name(),$sformatf( " Recived transaction imp port get request" ) , UVM_LOW ) trans = transaction : : type_id : : create ( "trans" , this ); void ' ( trans. randomize ( ) ); `uvm_info(get_type_name(),$sformatf( " tranaction randomized" ) , UVM_LOW ) `uvm_info(get_type_name(),$sformatf( " Printing trans, \n %s" , trans.sprint ( ) ) , UVM_LOW ) `uvm_info(get_type_name(),$sformatf( " Sendting trans packet" ) , UVM_LOW ) return 1; endfunction //--------------------------------------- // Imp port can_get method //--------------------------------------- //Setp-4: can_get() method. virtual function bit can_get ( ); endfunction endclass : component_a |
Note:
As the port is nonblocking, methods should be implemented as function not task.
* Implementing and using of can_put is shown in the next example
Connecting TLM Port and Imp Port in env
function void connect_phase ( uvm_phase phase ); comp_b.trans_in.connect ( comp_a.trans_out ); //Connecting the ports endfunction : connect_phase |
Simulator Output
UVM_INFO @ 0: reporter [RNTST] Running test basic_test... ------------------------------------------------------ Name Type Size Value ------------------------------------------------------ uvm_test_top basic_test - @335 env environment - @348 comp_a component_a - @357 trans_out uvm_nonblocking_get_imp - @366 comp_b component_b - @376 trans_in uvm_nonblocking_get_port - @385 ------------------------------------------------------ UVM_INFO component_b.sv(26) @ 0: uvm_test_top.env.comp_b [component_b] Requesting transaction. UVM_INFO component_b.sv(28) @ 0: uvm_test_top.env.comp_b [component_b] Before calling port get method UVM_INFO component_a.sv(24) @ 0: uvm_test_top.env.comp_a [component_a] Recived transaction imp port get request UVM_INFO component_a.sv(28) @ 0: uvm_test_top.env.comp_a [component_a] tranaction randomized UVM_INFO component_a.sv(29) @ 0: uvm_test_top.env.comp_a [component_a] Printing trans, --------------------------------- Name Type Size Value --------------------------------- trans transaction - @412 addr integral 4 'h3 wr_rd integral 1 'h1 wdata integral 8 'h6 --------------------------------- UVM_INFO component_a.sv(31) @ 0: uvm_test_top.env.comp_a [component_a] Sendting trans packet UVM_INFO component_b.sv(31) @ 0: uvm_test_top.env.comp_b [component_b] recived transaction from get method UVM_INFO component_b.sv(32) @ 0: uvm_test_top.env.comp_b [component_b] Printing trans, --------------------------------- Name Type Size Value --------------------------------- trans transaction - @412 addr integral 4 'h3 wr_rd integral 1 'h1 wdata integral 8 'h6 --------------------------------- UVM_INFO component_b.sv(37) @ 0: uvm_test_top.env.comp_b [component_b] After calling port get method UVM_INFO /apps/vcsmx/etc/uvm-1.2/src/base/uvm_objection.svh(1270) @ 0: reporter [TEST_DONE] UVM_INFO /apps/vcsmx/etc/uvm-1.2/src/base/uvm_report_server.svh(847) @ 0: reporter [UVM/REPORT/SERVER]
The next example shows the implementation of the try_put() method.
❮ Previous Next ❯