Multi Subscribers with Multiports
Table of Contents
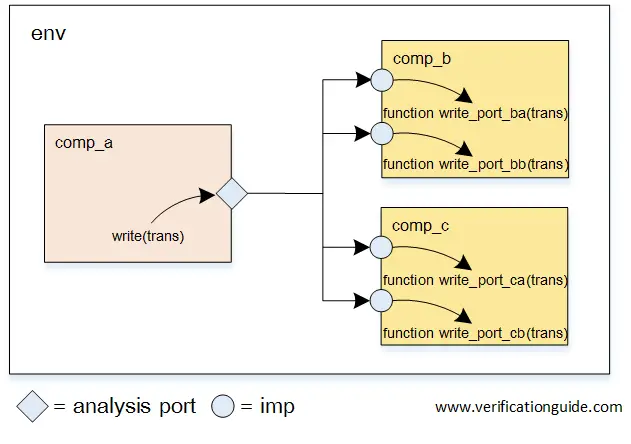
This example shows connecting the same analysis port to analysis imp ports of multiple components.
TLM Analysis TesetBench Components are,
——————————————————————-
Name Type
——————————————————————-
uvm_test_top basic_test
env environment
comp_a component_a
analysis_port uvm_analysis_port
comp_b component_b
analysis_imp_a uvm_analysis_imp_port_ba
analysis_imp_b uvm_analysis_imp_port_bb
comp_c component_c
analysis_imp_a uvm_analysis_imp_port_ca
analysis_imp_b uvm_analysis_imp_port_cb
——————————————————————-
Implementing analysis port in comp_a
class component_a extends uvm_component; transaction trans; //Step-1. Declaring analysis port uvm_analysis_port# ( transaction ) analysis_port; `uvm_component_utils( component_a ) //--------------------------------------- // Constructor //--------------------------------------- function new ( string name , uvm_component parent ); super . new ( name , parent ); //Step-2. Creating analysis port analysis_port = new ( "analysis_port" , this ); endfunction : new //--------------------------------------- // run_phase //--------------------------------------- virtual task run_phase ( uvm_phase phase ); phase.raise_objection ( this ); trans = transaction : : type_id : : create ( "trans" , this ); void ' ( trans. randomize ( ) ); `uvm_info(get_type_name(),$sformatf( " tranaction randomized" ) , UVM_LOW ) `uvm_info(get_type_name(),$sformatf( " Printing trans, \n %s" , trans.sprint ( ) ) , UVM_LOW ) `uvm_info(get_type_name(),$sformatf( " Before calling port write method" ) , UVM_LOW ) //Ste-3. Calling write method analysis_port.write ( trans ); `uvm_info(get_type_name(),$sformatf( " After calling port write method" ) , UVM_LOW ) phase.drop_objection ( this ); endtask : run_phase endclass : component_a |
Implementing analysis imp_port’s in comp_b
//Step-1. Define analysis imp ports `uvm_analysis_imp_decl( _port_ba ) `uvm_analysis_imp_decl( _port_bb ) class component_b extends uvm_component; transaction trans; //Step-2. Declare the analysis imp ports uvm_analysis_imp_port_ba # ( transaction , component_b ) analysis_imp_a; uvm_analysis_imp_port_bb # ( transaction , component_b ) analysis_imp_b; `uvm_component_utils( component_b ) //--------------------------------------- // Constructor //--------------------------------------- function new ( string name , uvm_component parent ); super . new ( name , parent ); //Step-3. Create the analysis imp ports analysis_imp_a = new ( "analysis_imp_a" , this ); analysis_imp_b = new ( "analysis_imp_b" , this ); endfunction : new //Step-4. Implement the write method write_port_ba //--------------------------------------- // Analysis port write method //--------------------------------------- virtual function void write_port_ba ( transaction trans ); `uvm_info(get_type_name(),$sformatf( " Inside write_port_ba method. Received trans On Analysis Imp Port" ) , UVM_LOW ) `uvm_info(get_type_name(),$sformatf( " Printing trans, \n %s" , trans.sprint ( ) ) , UVM_LOW ) endfunction //Step-4. Implement the write method write_port_bb //--------------------------------------- // Analysis port write method //--------------------------------------- virtual function void write_port_bb ( transaction trans ); `uvm_info(get_type_name(),$sformatf( " Inside write_port_bb method. Received trans On Analysis Imp Port" ) , UVM_LOW ) `uvm_info(get_type_name(),$sformatf( " Printing trans, \n %s" , trans.sprint ( ) ) , UVM_LOW ) endfunction endclass : component_b |
Implementing analysis imp_port’s in comp_c
//Step-1. Define analysis imp ports `uvm_analysis_imp_decl( _port_ca ) `uvm_analysis_imp_decl( _port_cb ) class component_c extends uvm_component; transaction trans; //Step-2. Declare the analysis imp ports uvm_analysis_imp_port_ca # ( transaction , component_c ) analysis_imp_a; uvm_analysis_imp_port_cb # ( transaction , component_c ) analysis_imp_b; `uvm_component_utils( component_c ) //--------------------------------------- // Constructor //--------------------------------------- function new ( string name , uvm_component parent ); super . new ( name , parent ); //Step-3. Create the analysis imp ports analysis_imp_a = new ( "analysis_imp_a" , this ); analysis_imp_b = new ( "analysis_imp_b" , this ); endfunction : new //Step-4. Implement the write method write_port_ca //--------------------------------------- // Analysis port write method //--------------------------------------- virtual function void write_port_ca ( transaction trans ); `uvm_info(get_type_name(),$sformatf( " Inside write_port_ba method. Received trans On Analysis Imp Port" ) , UVM_LOW ) `uvm_info(get_type_name(),$sformatf( " Printing trans, \n %s" , trans.sprint ( ) ) , UVM_LOW ) endfunction //Step-4. Implement the write method write_port_cb //--------------------------------------- // Analysis port write method //--------------------------------------- virtual function void write_port_cb ( transaction trans ); `uvm_info(get_type_name(),$sformatf( " Inside write_port_bb method. Received trans On Analysis Imp Port" ) , UVM_LOW ) `uvm_info(get_type_name(),$sformatf( " Printing trans, \n %s" , trans.sprint ( ) ) , UVM_LOW ) endfunction endclass : component_c |
Connecting analysis port and analysis imp_ports in env
function void connect_phase ( uvm_phase phase ); //Connecting analysis port to imp port comp_a.analysis_port.connect ( comp_b.analysis_imp_a ); comp_a.analysis_port.connect ( comp_b.analysis_imp_b ); comp_a.analysis_port.connect ( comp_c.analysis_imp_a ); comp_a.analysis_port.connect ( comp_c.analysis_imp_b ); endfunction : connect_phase |
Simulator Output
UVM_INFO @ 0: reporter [RNTST] Running test basic_test... ----------------------------------------------------------- Name Type Size Value ----------------------------------------------------------- uvm_test_top basic_test - @1842 env environment - @1911 comp_a component_a - @1943 analysis_port uvm_analysis_port - @1978 comp_b component_b - @2011 analysis_imp_a uvm_analysis_imp_port_ba - @2046 analysis_imp_b uvm_analysis_imp_port_bb - @2081 comp_c component_c - @2112 analysis_imp_a uvm_analysis_imp_port_ca - @2147 analysis_imp_b uvm_analysis_imp_port_cb - @2182 ----------------------------------------------------------- UVM_INFO component_a.sv(29) @ 0: uvm_test_top.env.comp_a [component_a] tranaction randomized UVM_INFO component_a.sv(30) @ 0: uvm_test_top.env.comp_a [component_a] Printing trans, --------------------------------- Name Type Size Value --------------------------------- trans transaction - @2235 addr integral 4 'he wr_rd integral 1 'h0 wdata integral 8 'h4 --------------------------------- UVM_INFO component_a.sv(32) @ 0: uvm_test_top.env.comp_a [component_a] Before calling port write method UVM_INFO component_b.sv(29) @ 0: uvm_test_top.env.comp_b [component_b] Inside write_port_ba method. Recived trans On Analysis Imp Port UVM_INFO component_b.sv(30) @ 0: uvm_test_top.env.comp_b [component_b] Printing trans, --------------------------------- Name Type Size Value --------------------------------- trans transaction - @2235 addr integral 4 'he wr_rd integral 1 'h0 wdata integral 8 'h4 --------------------------------- UVM_INFO component_b.sv(37) @ 0: uvm_test_top.env.comp_b [component_b] Inside write_port_bb method. Recived trans On Analysis Imp Port UVM_INFO component_b.sv(38) @ 0: uvm_test_top.env.comp_b [component_b] Printing trans, --------------------------------- Name Type Size Value --------------------------------- trans transaction - @2235 addr integral 4 'he wr_rd integral 1 'h0 wdata integral 8 'h4 --------------------------------- UVM_INFO component_c.sv(29) @ 0: uvm_test_top.env.comp_c [component_c] Inside write_port_ca method. Recived trans On Analysis Imp Port UVM_INFO component_c.sv(30) @ 0: uvm_test_top.env.comp_c [component_c] Printing trans, --------------------------------- Name Type Size Value --------------------------------- trans transaction - @2235 addr integral 4 'he wr_rd integral 1 'h0 wdata integral 8 'h4 --------------------------------- UVM_INFO component_c.sv(37) @ 0: uvm_test_top.env.comp_c [component_c] Inside write_port_cb method. Recived trans On Analysis Imp Port UVM_INFO component_c.sv(38) @ 0: uvm_test_top.env.comp_c [component_c] Printing trans, --------------------------------- Name Type Size Value --------------------------------- trans transaction - @2235 addr integral 4 'he wr_rd integral 1 'h0 wdata integral 8 'h4 --------------------------------- UVM_INFO component_a.sv(34) @ 0: uvm_test_top.env.comp_a [component_a] After calling port write method UVM_INFO /playground_lib/uvm-1.2/src/base/uvm_objection.svh(1271) @ 0: reporter [TEST_DONE] UVM_INFO /playground_lib/uvm-1.2/src/base/uvm_report_server.svh(847) @ 0: reporter [UVM/REPORT/SERVER]