Connecting multiple ports to a single analysis port
Table of Contents
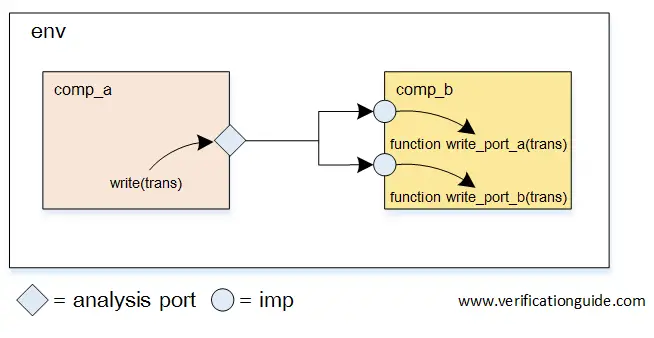
This example shows connecting analysis port to multiple analysis imp ports.
TLM Analysis ports TesetBench Components are,
——————————————————————-
Name Type
——————————————————————-
uvm_test_top basic_test
env environment
comp_a component_a
analysis_port uvm_analysis_port
comp_b component_b
analysis_imp_a uvm_analysis_imp_port_a
analysis_imp_b uvm_analysis_imp_port_b
——————————————————————-
Implementing analysis port in comp_a
class component_a extends uvm_component; transaction trans; //Step-1. Declaring analysis port uvm_analysis_port# ( transaction ) analysis_port; `uvm_component_utils( component_a ) //--------------------------------------- // Constructor //--------------------------------------- function new ( string name , uvm_component parent ); super . new ( name , parent ); //Step-2. Creating analysis port analysis_port = new ( "analysis_port" , this ); endfunction : new //--------------------------------------- // run_phase //--------------------------------------- virtual task run_phase ( uvm_phase phase ); phase.raise_objection ( this ); trans = transaction : : type_id : : create ( "trans" , this ); void ' ( trans. randomize ( ) ); `uvm_info(get_type_name(),$sformatf( " tranaction randomized" ) , UVM_LOW ) `uvm_info(get_type_name(),$sformatf( " Printing trans, \n %s" , trans.sprint ( ) ) , UVM_LOW ) `uvm_info(get_type_name(),$sformatf( " Before calling port write method" ) , UVM_LOW ) //Ste-3. Calling write method analysis_port.write ( trans ); `uvm_info(get_type_name(),$sformatf( " After calling port write method" ) , UVM_LOW ) phase.drop_objection ( this ); endtask : run_phase endclass : component_a |
Implementing analysis imp_ports in comp_b
Implementing analysis imp_ports in a component involves below steps,
1. define the analysis_imp_ports using macro
`uvm_analysis_imp_decl( port_identifier ) //port_identifier is the user defined name to differentiate the imp ports |
defining ports with the name _port_a and _port_b
`uvm_analysis_imp_decl( _port_a ) `uvm_analysis_imp_decl( _port_b ) |
2. Declare the analysis ports
uvm_analysis_imp<> # ( t , T ) port_name; |
declaring two ports using the above syntax,
uvm_analysis_imp_port_a # ( transaction , component_b ) analysis_imp_a; uvm_analysis_imp_port_b # ( transaction , component_b ) analysis_imp_b; |
3. Implement the write methods for each port,
function void write_port_identifier; |
Implementing write methods with the above syntax,
Method for analysis_imp_a
virtual function void write_port_a ( transaction trans ); `uvm_info(get_type_name(),$sformatf( " Inside write_port_a method. Received trans On Analysis Imp Port" ) , UVM_LOW ) `uvm_info(get_type_name(),$sformatf(" Printing trans, \n %s",trans.sprint( ) ) , UVM_LOW ) endfunction |
Method for analysis_imp_b
virtual function void write_port_b ( transaction trans ); `uvm_info(get_type_name(),$sformatf( " Inside write_port_b method. Received trans On Analysis Imp Port" ) , UVM_LOW ) `uvm_info(get_type_name(),$sformatf(" Printing trans, \n %s",trans.sprint( ) ) , UVM_LOW ) endfunction |
Complete comp_b code,
`uvm_analysis_imp_decl( _port_a ) `uvm_analysis_imp_decl( _port_b ) class component_b extends uvm_component; transaction trans; uvm_analysis_imp_port_a # ( transaction , component_b ) analysis_imp_a; uvm_analysis_imp_port_b # ( transaction , component_b ) analysis_imp_b; `uvm_component_utils( component_b ) //--------------------------------------- // Constructor //--------------------------------------- function new ( string name , uvm_component parent ); super . new ( name , parent ); analysis_imp_a = new ( "analysis_imp_a" , this ); analysis_imp_b = new ( "analysis_imp_b" , this ); endfunction : new //--------------------------------------- // Analysis port write method //--------------------------------------- virtual function void write_port_a ( transaction trans ); `uvm_info(get_type_name(),$sformatf( " Inside write_port_a method. Recived trans On Analysis Imp Port" ) , UVM_LOW ) `uvm_info(get_type_name(),$sformatf( " Printing trans, \n %s" , trans.sprint ( ) ) , UVM_LOW ) endfunction //--------------------------------------- // Analysis port write method //--------------------------------------- virtual function void write_port_b ( transaction trans ); `uvm_info(get_type_name(),$sformatf( " Inside write_port_b method. Recived trans On Analysis Imp Port" ) , UVM_LOW ) `uvm_info(get_type_name(),$sformatf( " Printing trans, \n %s" , trans.sprint ( ) ) , UVM_LOW ) endfunction endclass : component_b |
Connecting Analysis port with the imp_ports in env
function void connect_phase ( uvm_phase phase ); //Connecting analysis_port to imp_ports comp_a.analysis_port.connect ( comp_b.analysis_imp_a ); comp_a.analysis_port.connect ( comp_b.analysis_imp_b ); endfunction : connect_phase |
Simulator Output
UVM_INFO @ 0: reporter [RNTST] Running test basic_test... ---------------------------------------------------------- Name Type Size Value ---------------------------------------------------------- uvm_test_top basic_test - @1839 env environment - @1908 comp_a component_a - @1940 analysis_port uvm_analysis_port - @1975 comp_b component_b - @2008 analysis_imp_a uvm_analysis_imp_port_a - @2043 analysis_imp_b uvm_analysis_imp_port_b - @2078 ---------------------------------------------------------- UVM_INFO component_a.sv(29) @ 0: uvm_test_top.env.comp_a [component_a] tranaction randomized UVM_INFO component_a.sv(30) @ 0: uvm_test_top.env.comp_a [component_a] Printing trans, --------------------------------- Name Type Size Value --------------------------------- trans transaction - @209 addr integral 4 'he wr_rd integral 1 'h0 wdata integral 8 'h4 --------------------------------- UVM_INFO component_a.sv(32) @ 0: uvm_test_top.env.comp_a [component_a] Before calling port write method UVM_INFO component_b.sv(29) @ 0: uvm_test_top.env.comp_b [component_b] Inside write_port_a method. Recived trans On Analysis Imp Port UVM_INFO component_b.sv(30) @ 0: uvm_test_top.env.comp_b [component_b] Printing trans, --------------------------------- Name Type Size Value --------------------------------- trans transaction - @209 addr integral 4 'he wr_rd integral 1 'h0 wdata integral 8 'h4 --------------------------------- UVM_INFO component_b.sv(37) @ 0: uvm_test_top.env.comp_b [component_b] Inside write_port_b method. Recived trans On Analysis Imp Port UVM_INFO component_b.sv(38) @ 0: uvm_test_top.env.comp_b [component_b] Printing trans, --------------------------------- Name Type Size Value --------------------------------- trans transaction - @209 addr integral 4 'he wr_rd integral 1 'h0 wdata integral 8 'h4 --------------------------------- UVM_INFO component_a.sv(34) @ 0: uvm_test_top.env.comp_a [component_a] After calling port write method UVM_INFO /playground_lib/uvm-1.2/src/base/uvm_objection.svh(1271) @ 0: reporter [TEST_DONE] UVM_INFO /playground_lib/uvm-1.2/src/base/uvm_report_server.svh(847) @ 0: reporter [UVM/REPORT/SERVER]