UVM TLM Methods
Table of Contents
The TLM Interface class declares all the methods required to perform communication.
put
- put method is used to send a transaction to another component
- Calling <port>.put(trans) sends a transaction to other component
- put() method call is a blocking call. It will not return until the transaction has been successfully sent
get
- get method is used to retrieve transaction from another component
- Calling <port>.get(trans) retrieve transaction from other component
- get() method call is a blocking call. It will not return until the transaction has been successfully retrieved
- get() method call consumes the transaction. i.e like how FIFO get method will takeout the entry from FIFO
- Subsequent calls to get must return a different transaction instance. Means as the previous get will takeout the trans, new trans is expected
peek
- peek method obtain a transaction without consuming it
- Calling <port>.peek(trans) retrieve transaction from other component
- peek() method call is a blocking call.
- The returned transaction is not consumed. A subsequent peek or get will return the same transaction
try_put
- the try_put method is used to send a transaction to another component without blocking the execution
- Calling <port>.try_put(trans) sends a transaction to another component, if possible
- try_put method returns 1 If the component is ready to accept the transaction, otherwise it returns 0
can_put
- can_put() method call returns 1 if the component is ready to accept the transaction, otherwise, it returns 0
- no argument must be passed to can_put, this method is used to see whether the other component is ready to accept the trans
try_get
- the try_get method is used to retrieve transaction from another component without blocking the execution
- Calling <port>.try_get(trans) retrieve transaction from another component, if possible
- try_get method returns 1 if the transaction is available, otherwise, it returns 0
can_get
- can_get() method call returns 1 if the component can get transaction immediately, otherwise, it returns 0
- no argument must be passed to can_get, this method is used to see whether any transaction is available to get
transport
- Calling <port>.transport(req,resp) method executes the given request and returns the response in the given output argument
- The transport method call is blocking, it may block until the operation is complete
nb_transport
- Calling <port>.nb_transport(req,resp) the method executes the given request and returns the response in the given output argument, if possible
- If for any reason the operation could not be executed immediately, then a 0 must be returned, otherwise 1
Analysis
Write
- Calling <port>.write(trans) method will broadcasts a transaction to any number of listeners.
- Write method call is a nonblocking call
As along with the interface methods, TLM ports are required for the communication between the components, Usage of these methods is described after knowing about ports and exports.
Summary of UVM TLM Interface
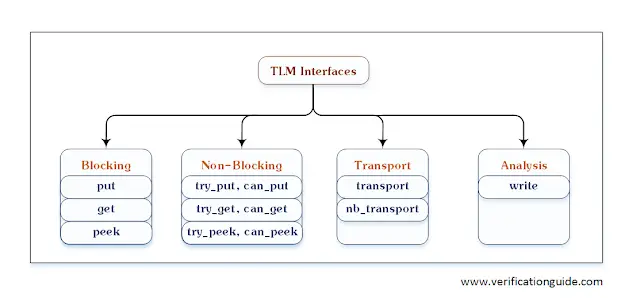